- Why Error Handling Matters
- The Go Philosophy on Error Handling
- Core Error Handling Techniques
- Advanced Error Handling Patterns
- Context and Error Propagation
- Logging and Monitoring Errors
- Error Handling in Concurrency
- Testing Error Handling
- Best Practices for Production Systems
- Conclusion
Tip: Effective error handling improves the reliability and maintainability of your applications, ensuring a seamless user experience. By helping developers understand the "why" behind each approach, we foster better adoption and cleaner implementations.
Why Error Handling Matters
Error handling is essential to building reliable applications. Properly managing errors ensures:
- Application Stability: Prevent crashes and undefined behavior.
- Maintainable Code: Create clear, consistent error-handling mechanisms.
- Actionable Debugging: Provide developers with meaningful insights for debugging.
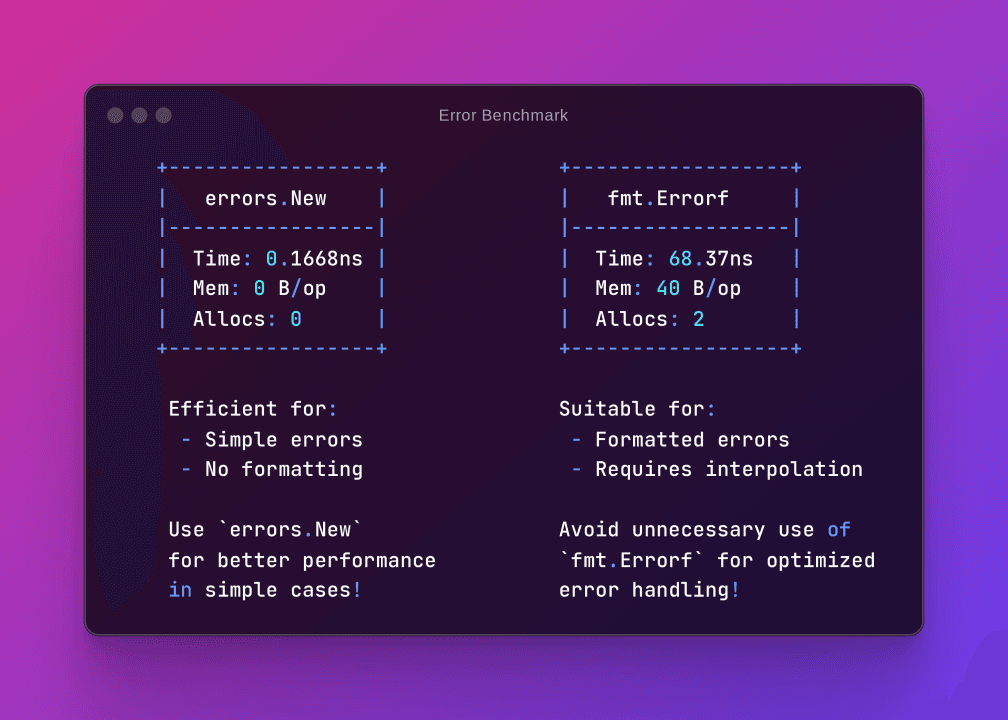
Understanding this flow ensures developers know where to focus their efforts during development.
The Go Philosophy on Error Handling
Go's error handling philosophy emphasizes simplicity and clarity:
- Errors as Values: Treat errors as regular values, allowing structured and testable handling. This approach ensures developers maintain control over their error handling, unlike languages with exceptions that may propagate silently.
- Explicit Control Flow: Avoid exceptions, preferring explicit checks for errors. This ensures clarity in how the program handles unexpected situations.
- Contextual Clarity: Provide sufficient context for errors without over-complicating the logic. This allows developers to quickly trace and resolve issues.
Core Error Handling Techniques
Returning Errors
The most fundamental pattern involves returning errors alongside results. This approach:
- Makes the control flow explicit.
- Encourages developers to address errors immediately.
Example:
func readFile(filename string) ([]byte, error) {
data, err := os.ReadFile(filename)
if err != nil {
return nil, err
}
return data, nil
}
Wrapping Errors with fmt.Errorf
Add context to errors for better debugging. For example:
func readFile(filename string) ([]byte, error) {
data, err := os.ReadFile(filename)
if err != nil {
return nil, fmt.Errorf("failed to read file %s: %w", filename, err)
}
return data, nil
}
This ensures that developers have meaningful error messages, especially when logs are the first point of investigation.
Using the errors
Package
Go's errors
package includes utilities like errors.Is
and errors.As
for error introspection. This allows developers to handle specific error types cleanly:
if errors.Is(err, os.ErrNotExist) {
log.Println("File does not exist")
}
By structuring error checking this way, developers can provide specific error-handling strategies without ambiguous or generic fallbacks.
Advanced Error Handling Patterns
Custom Error Types
Define custom error types for structured error information. This allows developers to include meaningful metadata about the error:
type ValidationError struct {
Field string
Msg string
}
func (e ValidationError) Error() string {
return fmt.Sprintf("validation error on %s: %s", e.Field, e.Msg)
}
This approach is particularly useful in APIs, where returning detailed error information improves the developer experience.
Error Sentinels
Use predefined errors for specific scenarios. For instance:
var ErrUnauthorized = errors.New("unauthorized access")
func authenticate(user string) error {
if user != "admin" {
return ErrUnauthorized
}
return nil
}
Sentinels provide a clear contract to other developers using your code, defining known error scenarios explicitly.
Error Aggregation
Aggregate multiple errors for batch processing:
type MultiError struct {
Errors []error
}
func (m MultiError) Error() string {
msgs := []string{}
for _, err := range m.Errors {
msgs = append(msgs, err.Error())
}
return strings.Join(msgs, "; ")
}
This pattern is particularly useful for batch processing jobs where multiple issues might arise.
Decorating Errors
Enhance error messages dynamically, guiding developers on what went wrong:
func DecorateError(err error, msg string) error {
return fmt.Errorf("%s: %w", msg, err)
}
This approach provides richer context without creating new error types, balancing flexibility with simplicity.
Context and Error Propagation
Use the context
package to propagate cancellation or timeouts. This ensures developers can manage application state gracefully:
func fetchData(ctx context.Context, url string) (string, error) {
req, err := http.NewRequestWithContext(ctx, "GET", url, nil)
if err != nil {
return "", err
}
resp, err := http.DefaultClient.Do(req)
if err != nil {
return "", err
}
defer resp.Body.Close()
body, err := io.ReadAll(resp.Body)
if err != nil {
return "", err
}
return string(body), nil
}
Logging and Monitoring Errors
Leverage structured logging and monitoring tools to capture and analyze errors:
logger, _ := zap.NewProduction()
defer logger.Sync()
logger.Error("an error occurred", zap.Error(err))
Include a diagram showing how errors flow through logging and monitoring systems, emphasizing key points for troubleshooting.
Error Handling in Concurrency
Handle errors in goroutines with channels. This ensures errors are not lost during concurrent execution:
func worker(errors chan error) {
if err := doTask(); err != nil {
errors <- err
}
}
go func() {
errors := make(chan error)
go worker(errors)
if err := <-errors; err != nil {
log.Println("Worker failed:", err)
}
}()
Providing this structure allows developers to maintain control and insight into concurrent processes.
Testing Error Handling
Write unit tests to cover error scenarios. Emphasize the importance of covering edge cases:
func TestReadFile_Error(t *testing.T) {
_, err := readFile("nonexistent.txt")
if !errors.Is(err, os.ErrNotExist) {
t.Errorf("expected os.ErrNotExist, got %v", err)
}
}
Mock dependencies to simulate critical failure points.
Best Practices for Production Systems
- Centralized Logging: Aggregate logs from distributed systems.
- Consistent Patterns: Establish and enforce error-handling conventions.
- Document Errors: Maintain an error catalog for easier debugging.
- Graceful Recovery: Use
recover
sparingly to handle panics in critical sections.
By sharing practical examples, developers can adopt these practices effectively in their workflows.
Conclusion
Effective error handling is critical for building robust and maintainable Go applications. By adopting structured patterns, leveraging advanced tools, and integrating testing and monitoring, you can create a codebase that is resilient and developer-friendly. Implement these strategies today to take your Go development to the next level.